Uninformed Search
- One of the oldest AI techniques
- Pioneered by Newell and Simon (Logic Theorist, General Problem Solver)
- Central component of more recent problem-solving systems such as SOAR
Reading
- Textbook sections 3.1 - 4.11
- Supplementary reading #2: Solving Problems by
Searching, by Russell and Norvig
Terminology
- world states
- states and state space
- initial state
- operators (or actions)
- successor function
- step cost and path cost
- goal state
- goal test
- problem: initial state, successor function, goal test, path cost function
- search: find an operator sequence that moves agent from initial state to a
goal state
Examples of Problem Formulation
Problem-formulation is the most important step in creating a successful
search application. How to choose an appropriate level of abstraction for
states and operators?
Route planning
- states: individual cities
- operators: driving from one city to the next
- goal: a particular city
- step cost: time, distance, fuel, danger, etc.
Eight-puzzle
- states: tile configurations
- operators: sliding one tile up/down/left/right
- goal: all tiles in some desired order
- step cost: 1
Map coloring
- states: assignments of colors to regions
- operators: assign a color to an uncolored region that does not conflict with other regions
- goal: all regions colored
- step cost: 0
Cryptarithmetic
- states: puzzle with some letters replaced by digits
- operators: replace a letter with a digit
- goal: no letters left, correct sum
- example:
CATS 6154 (C=6, A=1, T=5, S=4, E=9, M=7, I=0)
+ EAT + 915
------ -----
MICE 7069
- step cost: 0
Missionaries and cannibals
- states: number of missionaries and cannibals on each side of river, location of boat
- operators: take 1 or 2 people across the river in the boat without leaving
more cannibals than missionaries on one side
- initial state: everyone on left side, boat on left
- goal state: everyone on right side, boat on right
- step cost: 1
Water jug puzzle
- states: amount of water in 3-quart pitcher and 4-quart pitcher
- operators:
- fill up either pitcher from the faucet
- pour all water from either pitcher down the drain
- pour water from one pitcher to the other, up to the brim
- initial state: both pitchers empty
- goal state: 3-quart pitcher empty; exactly 2 quarts left in 4-quart pitcher
- step cost: 1
Uninformed Search Strategies
No information about how far we are from a goal state.
Different strategies answer the following questions in different ways:
- Are we guaranteed to find a solution if one exists? (completeness)
- Will the optimal solution always be found? (optimality)
- How long will it take? (time complexity)
- How much memory is needed? (space complexity)
General search algorithm:
SEARCH(problem, strategy) {
create a search tree with the initial state at the root
repeat {
if there are no candidates for expansion
return failure
choose a leaf node for expansion according to strategy
if the node represents a goal state
return solution
else
generate successors and add them to the candidate list
}
}
|
Keep in mind:
Distinction between states of state space and nodes of search tree
States describe features of the world
Nodes include states, but also lots of other information:
- state
- depth of node in the tree
- parent node
- cost of the path so far, usually denoted g(n)
- action that generated this node
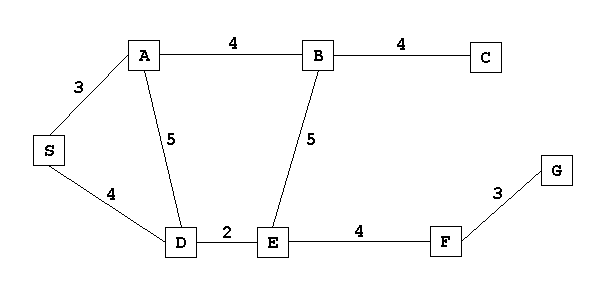
Breadth-first search
- expands shallowest node next
- pushes forward uniformly into the search tree
- complete
- optimal (if path cost is a nondecreasing function of depth)
- candidate list is an ordinary queue
- exponential in time and space
Uniform-cost search
- expands least-cost node next
- complete
- optimal (if steps costs are non-negative)
- candidate list is a priority queue ordered by path cost
- exponential in time and space
- same as breadth-first search if step costs are all equal
Nondeterministic search
- expands random node next
- not complete
- not optimal
- candidate list is a randomized set
- exponential in time and space
- can be useful when branching factor is large and long useless paths may exist
Depth-first search
- expands deepest node next
- dives headlong into the search tree
- not complete (may get sidetracked and never return)
- not optimal (may find suboptimal solution first)
- candidate list is a stack
- exponential in time
- efficient in space
- more efficient than breadth-first when many solutions exist
Depth-limited search
- expands deepest node next, up to a point
- complete (only if a solution exists within depth limit)
- not optimal
- exponential in time
- efficient in space
- unclear how to choose depth limit
Iterative-deepening search
- depth-limited search with increasing depth limits
- complete
- optimal
- exponential in time
- efficient in space
- expands some nodes multiple times
- multiple expansion overhead is not a serious problem
- preferred search method when the search space is large and
the depth of a solution is not known
Bidirectional search
- searches forward from initial state and backwards from goal
state simultaneously, using breadth-first search
- complete
- optimal
- exponential in time and space, but with better bounds:
O(bd/2) instead of O(bd)
- not applicable to every problem
- works best with single goal state and reversible operators
Summary of time and space complexities of search strategies is given on
page 81 of SR #2
Avoiding Repeated States
- Maintain a record of states previously encountered
- Such a record is usually called the closed list
SEARCH(problem, strategy) {
create a search tree with the initial state at the root
create an empty closed list
repeat {
if there are no candidates for expansion
return failure
choose a leaf node for expansion according to strategy
if this node's state is already in closed list
discard node and continue
else if the node represents a goal state
return solution
else
add node's state to closed list
generate successors and add them to the candidate list
}
}
|